Web services are a popular and well-understood way of
integrating applications that are deployed within an enterprise’s
perimeter, or intranet. Examples of such applications include enterprise
resource planning (ERP) applications, CRM applications, productivity
applications such as Microsoft Office, and so on.
Integrating applications with third-party Web
services over the Internet has also become viable and in many cases is
the preferred approach to quickly adding new functionality to complex
applications. Web services can range from simple address validation or
credit card checks to more-complex tax calculations or treasury
services. Integrating applications such as Dynamics AX with external Web
services is often referred to as Software-plus-Services (S+S).
The power of S+S lies in the opportunity to
leverage the functionality of (almost) any Web service that is available
as needed and thus in being able to outsource certain parts of business
processes to trusted, specialized service providers in a cost-efficient
way.
Dynamics AX Service References
Dynamics AX service references can help you
quickly integrate Dynamics AX with external Web services. Dynamics AX
service references are conceptually similar to the service references in
Microsoft Visual Studio 2008: each service reference represents a local
interface to a remote Web service. A local API, that is, a proxy,
can be used to communicate with the Web service. The proxy that is
generated with Dynamics AX service references consists of .NET artifacts
that can be discovered and used from X++ through CLR interop. Figure 7 illustrates how an external Web service can be integrated with Dynamics AX.
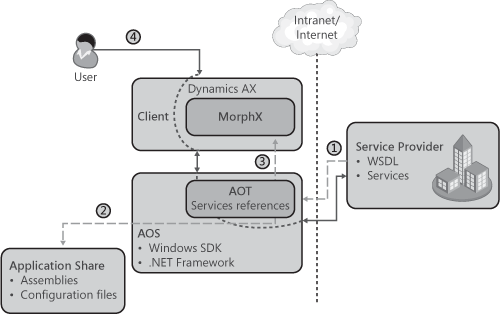
You generate Dynamics AX service references by
using standard Microsoft tools that are part of .NET Framework 3.5 and
Windows SDK for Windows Server 2008.
Consuming an External Web Service
In Dynamics AX, you can write code that consumes
external Web services fairly easily. You need to take these simple
steps at design time:
1. | Get the WSDL file for the external Web service.
|
2. | Create a Dynamics AX service reference.
|
3. | |
4. | Then
at run time, consume just the Web service as part of a business task
and configure the service references as needed. We go over these steps
in more detail in the following subsections.
|
Tip
Once you make sure Dynamics AX can connect to the external Web service, it is ready to go. |
Getting the WSDL and Creating a Service Reference
First, you need to get access to the WSDL file
for the service you want to consume. You can generate service references
from WSDL files that are available online (addressed through http or
https URLs) or offline in the file system (addressed through Universal
Naming Convention [UNC] file paths).
Tip
Make sure the service contract (WSDL file) hasn’t changed since the integration was implemented. |
To create a service reference, right-click the
AOT node References, select Add Service Reference, and enter four pieces
of information:
WSDL URL The (http or https) URL or UNC file path pointing to the WSDL file for the target Web service.
.NET code namespace Lets you discover the generated .NET artifacts with IntelliSense.
Reference name A unique name by which the service reference can be identified in the Dynamics AX client.
Description (optional) A short text description of the service reference.
Tip
If you get the error “Cannot load CLR Object” while creating a service reference at run time, restart the AOS. |
Once you fill in the information required for
creating the service reference and click OK, an AOT node representing
the new service reference is added.
Writing Integration Code
When the service reference is created, a .NET
proxy for the external Web service is generated. You can use this .NET
proxy from X++ code, through CLR interop, to communicate with the
external Web service. You must grant CLR interop permissions to the code
that accesses .NET assemblies to instantiate
parameters used for invoking an operation of an external Web service or
for consuming the service itself.
Use the .NET namespace you provided when you
created the service reference and IntelliSense to discover the generated
.NET artifacts from X++.
When you write code to consume external Web services from X++, you need to make sure it has the following characteristics:
It is marked to run on the server (AOS).
It grants and revokes interop permissions.
It
explicitly passes a WCF endpoint name into the proxy constructor. The
names of the available WCF endpoints are in the proxy’s WCF
configuration file (in the AIF administration form Service References,
click Configure).
It uses a valid license code for the external Web service (if applicable).
The following code sample uses the .NET proxy to consume the external Web service.
public static server boolean CheckCustomer (str customerName)
{
DynamicsOfac.OfacSoapClient c;
boolean b;
// grant interop permissions
new InteropPermission(InteropKind::ClrInterop).assert();
// instantiate proxy using WCF endpoint name
c = new DynamicsOfac.OfacSoapClient("OfacSoap");
// consume Dynamics OFAC Web service
b = c.LookupSdnByName(customerName);
// revoke interop permissions
CodeAccessPermission::revertAssert();
// return results
return b;
}
|
You need to write or modify code to use the
results returned by the external Web service: for example, validation
code that invokes a method similar to the one above that consumes an
external Web service and returns the results.
Configuring Service References
You can view a list of available service
references in the form Service References. To configure the WCF
parameters for the new service reference, click Configure. Keep in mind
that any changes you apply to the WCF configuration of the service proxy must be compatible with the WCF configuration of the service.
For more details on available WCF configuration parameters, see the Microsoft Dynamics AX 2009 SDK.
Note
To
configure Dynamics AX Services, we strongly recommend that you install
Windows SDK for Windows Server 2008 so you can leverage the WCF
configuration tools that are part of that SDK. |
Guidelines for Consuming External Web Services
The main driver for programmatically consuming external Web services is improved efficiency—both technically and economically.
Integration Guidelines
Web services are a means for machine-to-machine
integration. To streamline business processes, you should minimize the
user’s interaction with the external Web service. The consumption of
external Web services should always be in the background whenever
possible and involve the user only when additional input is needed. The
fact that the implementation of the business task consumes an external
Web service is an internal implementation detail that isn’t relevant to
the user.
When integrating external Web services, you
should try to avoid duplicate data entry, which is time-consuming and
error-prone. For example, assume that a sales order form has been filled
out and a Web service needs to be invoked to calculate the best
shipping option for delivering the goods to the recipient. It wouldn’t
make sense to launch a form that prompts for details such as delivery
address, weight, and so on. Instead, that information can be retrieved
from the sales order form, transparent to the user; other data, such as
weight, might be easily read from the database. Automatic data
correlation helps reduce the risks inherent in data entry.
You should integrate external Web services in a
way that allows you to switch service providers without affecting the
rest of your Dynamics AX application code. One way of achieving this is
through defining X++ interfaces for (relevant) functionality the
external Web service exposes. This switching capability also shields
your application logic from changes in the service contract (WSDL)
published by the service provider.
Security Guidelines
You need to be very careful in choosing your
service providers whenever you plan on exchanging confidential or
business-critical data. Service-level agreements with your service providers
should clearly define the provided security precautions as well as
other parameters that are necessary for your scenario, such as
guaranteed system uptime, response times, and so on. Be sure to conduct a
proper security review before you go live with integrations with
external Web services.
Refer to other literature or expert advice for more-complete information on securing integrations.
Privacy
When you exchange data with an external
system—especially when that external system is outside the perimeter of
your enterprise—you should always make sure that both data integrity and
privacy are guaranteed. Because it is the external service that
dictates the communication protocol and its configuration (including
security), you should consider the supported security mechanisms when
making a decision on what service provider to use for a particular
integration.
Authentication
Because the code that consumes the external
Web service is executed on the Dynamics AX server (AOS), AOS’s
identity—not the user’s identity—is used for authenticating requests to
external Web services. In most scenarios in which a third-party service
provides the external Web service, using AOS’s identity isn’t a
restriction. Authentication (and billing) would typically be per Web
service call per company instead of per user.
To prevent spoofing, we strongly recommend that you request server certificates for server authentication.
Authorization
When consuming external Web services that are
published by a third-party service provider, users who are authorized to
perform a business task should automatically be authorized to consume
all Web services needed to accomplish that task. For example, a user who
is authorized to create a new customer record should automatically be
authorized to use an external Web service for running a background
check. The user shouldn’t have to be explicitly authorized to consume
the Web service that performs the background check.
Custom SOAP Headers
If configured, WCF automatically inserts
standard SOAP headers (such as used for WS-Addressing) into messages.
Custom SOAP headers—that is, SOAP headers that are not understood by the
WCF stack—are not supported in Dynamics AX 2009. In other words, the
service proxies generated for service references don’t have an API for
setting SOAP headers programmatically.
Keep in mind that the use of custom SOAP
headers isn’t yet widely spread. Most commercial Web services don’t rely
on them to increase interoperability.